Table of Content
Backtrader | A Comprehensive Beginner’s Guide to Python Algorithmic Trading
By Vincent NguyenUpdated 47 days ago
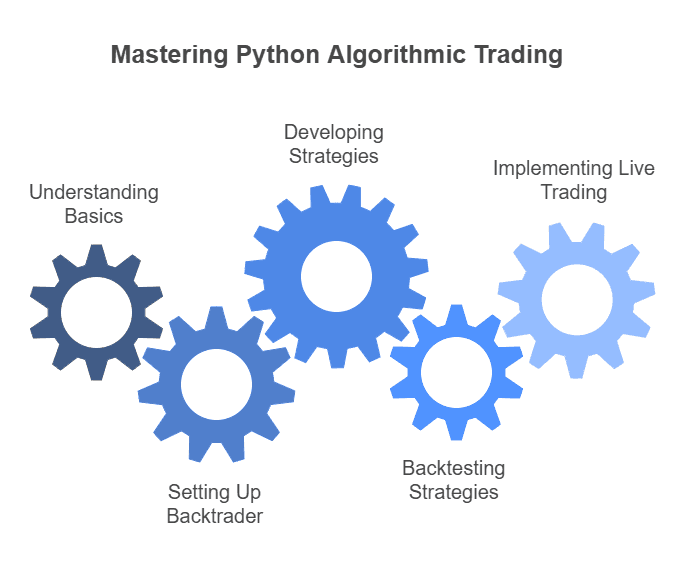
Getting Started with Backtrader: A Fast, Flexible Python Solution for Traders
Backtrader is an open-source Python library that helps you build, backtest, and optimize algorithmic trading strategies quickly. It uses an event-driven approach for analyzing financial markets, generating signals, and placing trades with minimal lines of code. Many traders rely on backtrader to test simple or advanced technical indicators, such as an average crossover strategy.
You can use built-in features or create reusable trading strategies to match any market conditions. In this full guide, you will learn basic functionality, important concepts, and practical steps for harnessing backtrader.
By the end, you’ll know how to backtest your strategies with cerebro=bt, interpret key metrics, and refine your approach.
Understanding the Basics of Backtrader
Backtrader is a versatile tool for both newcomers and seasoned trader enthusiasts. It serves as a Backtesting Framework that supports algorithmic trading strategies with minimal time building infrastructure. This open-source Python library runs on a simple architecture. It revolves around cerebro=bt, which acts as the engine for loading data feeds, executing orders, and compiling performance results.
How Backtrader Handles Data
Backtrader simplifies data management by letting you import pandas DataFrames, CSV files, or even live feeds. You can track the opening and closing price for each time period, as well as any technical indicators you wish to include. This helps you maintain a quick overview of market conditions without writing a lot of code blocks.
The Event-Driven Approach
At the core of backtrader lies an event-driven system. It processes new data and triggers strategy logic, allowing you to develop an effective strategy aligned with your trading goals. You can test an average crossover strategy or any other type of simple strategy by defining entry and exit criteria. Once backtested, you can refine your approach based on key performance metrics like maximum drawdown or net profit.
Key Components in Backtrader
- Strategies: Contain the rules, logic, and exit strategy details.
- Indicators: Measure market behavior, such as simple moving averages or average price levels.
- Analyzers: Evaluate performance, including potential risk, drawdown, and annualized metrics.
- Observers: Provide visual plots and logs of individual trades and portfolio values.
- Cerebro (bt): Orchestrates data, calculates trades, and consolidates results. Backtrader transforms algorithmic trading strategy development into a smoother process by focusing on these key components. You gain flexibility for analysis of trading strategies, faster iteration cycles, and more consistent results. Over time, this structure lets you adapt to shifting market conditions without rebuilding your entire codebase.
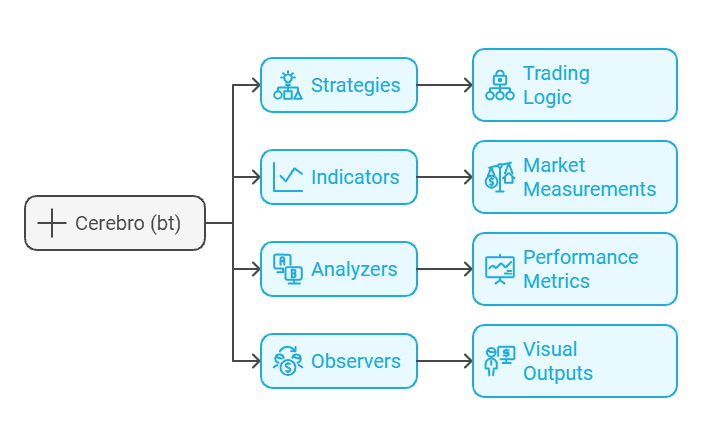
Setting Up Your Backtrader Environment
Backtrader runs on any system with Python installed. This open-source Python library also works smoothly with third-party library integrations, such as import yfinance or Interactive Brokers data feeds. You can begin by installing the core package through a simple pip command. Then, you can add built-in data feed options or more specialized sources if you want a comprehensive suite of market data.
Installing Backtrader
Open your terminal or command prompt. Type:
bash
Copy codepip install backtrader
This command will fetch and install the latest stable release from PyPI. The process takes only seconds. You will then have everything you need to start coding your first backtesting process.
Verifying the Installation
Launch a Python session and test your setup by importing backtrader:
python
Copy codeimport backtrader as bt
If it imports successfully, your environment is ready. You can also load a basic data sample for quick overview. That ensures you can receive closing price data and other relevant fields without errors.
Managing Dependencies
Although backtrader stands as a versatile tool on its own, many traders use additional functionality to improve their workflow. For instance, you can leverage import yfinance to pull daily data from Yahoo Finance. This helps you avoid spending too much time building infrastructure. Everything stays streamlined within backtrader’s Cerebro Engine, which handles tasks related to data feeds, individual trades, and strategy performance.
Configuring Your Initial Cash and Broker Settings
Backtrader lets you specify initial cash with just a few lines of code. You can modify your trade size, limit price, and other broker-related parameters. This level of control eases you into basic risk management without having to write hundreds of lines of code. Once configured, you can focus on defining your own exit strategy or analyzing your final POTENTIAL RISK and overall strategy performance
Creating Your First Strategy in Backtrader
Backtrader supports a simple approach to developing algorithmic trading strategies. You can define a basic backtesting setup in just a few lines of source code. This helps you focus on analysis of trading strategies instead of worrying about heavy infrastructure. One popular method is the SMA Crossover Strategy, which uses built-in indicators like moving averages to determine entry and exit signals.
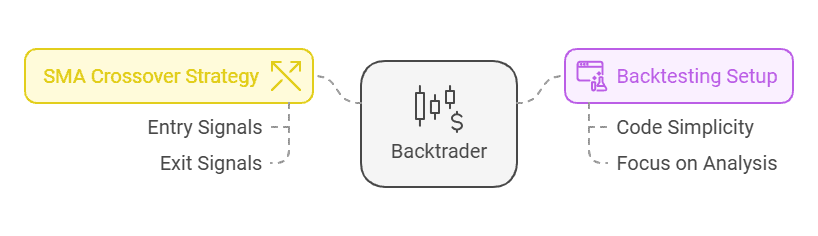
Why an Average Crossover Strategy?
A Crossover trading strategy detects bullish crossover points when a short-term average price surpasses a longer-term average price. It helps confirm potential upward momentum before placing trades. This average strategy can be effective in both trending and mixed market conditions, although it may generate false signals in choppy periods.
Defining the Strategy Class
Below is a quick code snippet that illustrates a simple structure:
python Copy code import backtrader as bt
class SmaCross(bt.Strategy): params = (('fast_period', 10), ('slow_period', 30),)
def __init__(self):
self.fast_sma = bt.ind.SMA(self.data.close, period=self.params.fast_period)
self.slow_sma = bt.ind.SMA(self.data.close, period=self.params.slow_period)
self.crossover = bt.ind.CrossOver(self.fast_sma, self.slow_sma)
def next(self):
if not self.position:
if self.crossover > 0:
self.buy()
elif self.crossover < 0:
self.close()
This class with methods for init() and next() shows how backtrader processes each bar of data. It compares two built-in indicators: a 10-day SMA and a 30-day SMA. When the fast SMA exceeds the slow SMA, the strategy issues a buy order. If it drops back under, the strategy closes that position.
Adding Your Strategy to Cerebro
To run this code, you link it to your Cerebro Engine:
python Copy code cerebro = bt.Cerebro() cerebro.addstrategy(SmaCross) This backtrader setup uses your defined strategy class as a backtesting platform. You can then load a data feed, specify the initial cash, and run the backtesting process. This simple strategy provides a quick overview of how to detect bullish crossover points. It also outlines how to place trades using your chosen exit strategy.
Analyzing the Results
Once you finish your backtesting process, you can examine individual trades, net profit, or potential risk. Backtrader analyzers provide key metrics like maximum drawdown. They also help you see if your strategy remains robust across different market regimes. By building on this average crossover strategy, you can experiment with additional parameters or test other technical indicators. That way, you can gradually evolve a profitable strategy aligned with your unique objectives.
Expanding Your Algorithmic Trading Strategies with Backtrader
Backtrader helps you scale beyond a simple strategy by offering robust tools for advanced analysis. You can monitor key components like drawdowns, Sharpe ratios, and other basic performance metrics through backtrader analyzers. These analytics highlight how your approach holds up under varying market regimes. They also guide you in making improvements without rewriting massive lines of code.
Using More Indicators and Multiple Feeds
You can easily add built-in indicators or a third-party library for specialized technical indicators. This flexibility lets you integrate everything from RSI to Bollinger Bands. Also, you can import multiple data sources and time periods. That approach allows you to test algorithmic trading strategy development across different assets multiple times, reducing the risk of overfitting a single market.
Leveraging Built-in Optimization
Three strategies examples to get started
Three strategies examples from the backtests library tradesearcher to try to implement with backtrader.
VIDYA Trend Strategy
Bitcoin / TetherUS (BTCUSDT)
@ 2 h
1.91
Risk Reward6,705.87 %
Total ROI4601
Total TradesTry Premium to view this strategy and 100K+ others.
Premium users can access all backtests with a Risk/Reward Ratio > 3
@ 1 h
3.69
Risk Reward684.55 %
Total ROI457
Total TradesBacktrader’s built-in optimization functionality explores parameter space automatically. It tries varying additional parameters for your moving averages, stop losses, or other strategy inputs. Then, it provides key performance metrics to help you compare each run. This means you can discover a more profitable strategy without manual tweaking of your exit strategy or fast and slow periods.
Real-World Deployment Considerations
Moving from the backtesting platform into actual market trading requires caution. Make sure your strategy evaluation includes various market conditions, liquidity constraints, and robust risk management. When your approach is stable, you can then hook up a broker connection. That way, you can transition from sample testing to live trading with minimal code changes.
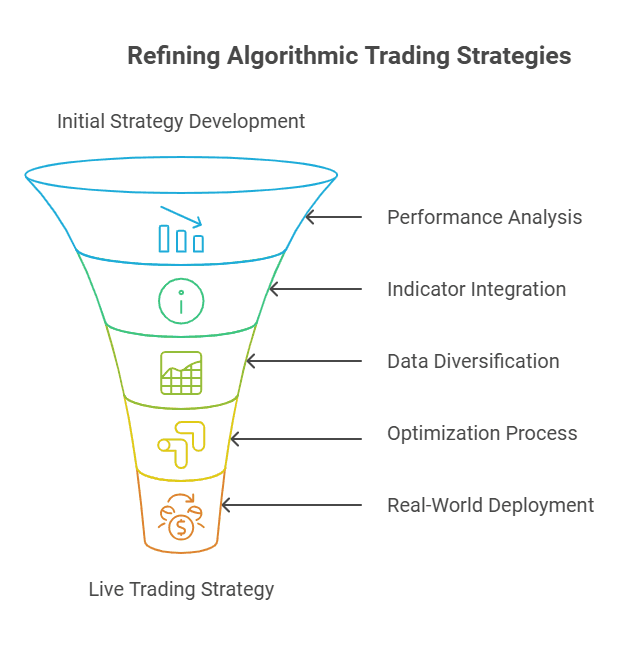
Performance Analysis and Visualization in Backtrader
Backtrader offers powerful tools for evaluating strategy performance, including built-in analyzers for drawdown, return ratios, and other key metrics. These features allow both new and advanced users to dive deep into quantitative analysis without getting lost in complex code. By leveraging the platform’s charting functionality and automation, you can conduct a detailed explanation of how well your algorithmic trading strategies respond to changing financial markets.
Leveraging Backtrader Analyzers
Built-in analyzers in backtrader function like modular plugins. They track portfolio values, maximum drawdown, and even annualized metrics over various time periods. You can set them up with minimal lines of code to receive insights about basic performance metrics. This analysis component guides your improvements, whether you’re refining a single average crossover strategy or managing multiple assets.
python
Copy code# Example of adding analyzers to your Cerebro Engine:
cerebro = bt.Cerebro()
cerebro.addanalyzer(bt.analyzers.SharpeRatio, _name='sharpe')
cerebro.addanalyzer(bt.analyzers.DrawDown, _name='drawdown')
By calling these analyzers, you’ll see if your exit strategy works under different market conditions. That information is crucial for effective strategy refinement and building a profitable strategy you can trust in actual market environments.
Visualizing Your Trading Results
Backtrader also supports visual plots for your backtests, including lines for trades, indicators, and equity curves. These easy-to-read graphics allow you to verify whether your logic holds up during random price fluctuations. They serve as a quick overview of each individual trade’s entry and exit, as well as your cumulative performance over time.
- Equity Curves: Show how your strategy’s total value changes after each trade.
- Indicator Overlays: Reveal how technical indicators, such as moving averages, align with your trades.
- Trade Annotations: Mark each executed order on the chart, so you can pinpoint missed opportunities or unwanted signals.
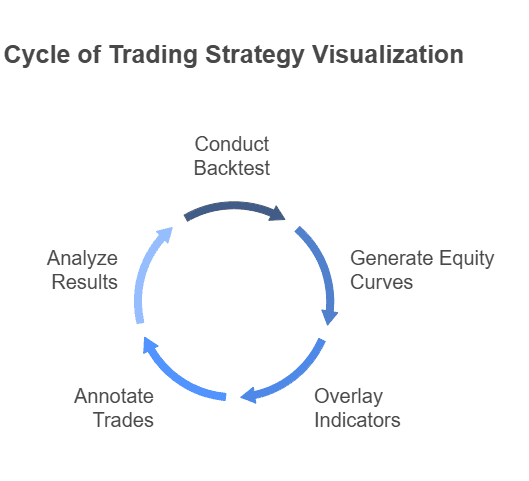
Exploring Advanced Visualizations
If you want more flexibility, you can integrate third-party libraries like matplotlib, Bokeh, or Plotly. While backtrader’s native charting functionality meets many needs, advanced users sometimes prefer separate tools for a 3D surface plot or specialized performance dashboards. These custom visuals can highlight areas where you need further strategy evaluation. Over time, you can reduce your POTENTIAL RISK by identifying recurring weaknesses.
Understanding Key Takeaways from Data Analysis
- Drawdown Patterns: Reveal how much of your capital you might lose during a trough decline.
- Trade-by-Trade Breakdown: Illustrates how each entry and exit contributed to gains or losses.
- Risk-to-Reward: Shows whether your approach delivers an effective strategy for long-term viability.
These insights prove invaluable as you refine your code, add new technical indicators, or incorporate additional parameters. The focus remains on systematic tweaks, not rewriting everything from scratch. Through consistent performance analysis, you can pivot or pause before stepping into live trading.
Practical Tips and Troubleshooting in Backtrader
Backtrader can sometimes throw you unexpected challenges, even if you follow a basic backtesting setup. Newcomers and advanced users alike must know how to handle common pitfalls to keep their algorithmic trading strategies running smoothly. Below are practical tips and troubleshooting steps to help you deal with typical issues in this open-source framework.
Common Data Alignment Issues
Data discrepancies often lead to inaccurate results. If your data feed has missing dates or odd time periods, backtrader might skip bars or misalign trades. To avoid these errors:
- Check for null values in your CSV or imported DataFrame.
- Make sure each row has a valid closing price, opening price, and timestamp.
- Align all time zones correctly, especially if you trade multiple assets.
Handling Attribute Errors
You might see an Attribute Error if you reference a function or field that doesn’t exist within backtrader’s core modules. This basic error occurs when you incorrectly spell a method or import. To resolve such issues:
- Verify that you spelled class names and function names correctly.
- Confirm that you’ve updated to the latest backtrader version.
- Check that your custom indicators or analyzers adhere to the base class feed or analyzer class structure.
Debugging Strategy Logic
Sometimes your base logic delivers unexpected trades or overlooks good entry points. Examine your strategy class by:
- Printing the fast and slow moving average values for a Crossover trading strategy to confirm they match your expectations.
- Logging trade size, order status, and potential limit price during each bar to identify where things go off track.
Reducing Memory Footprint
Processing large datasets can drain system memory. To streamline performance:
- Use a lower frequency (e.g., daily) rather than minute data when doing broad analysis of trading strategies.
- Limit the number of indicators in your code. Overloading with unneeded calculations might slow you down.
- Consider chunking your data into smaller segments if you face memory constraints.
Reevaluating Market Regimes
Market conditions are never static. A profitable strategy in one environment may fail when volatility spikes. Revisit your logic if you:
- Notice frequent whipsaws or steep drawdowns.
- See that your exit strategy triggers too late or too early under new trends.
- Want to adapt your technical indicators to more volatile market regimes.
By staying aware of these potential pitfalls and solutions, you will safeguard your backtrader workflows. This approach empowers you to build reusable trading strategies that handle a wider range of scenarios. Over time, you will gain insight into how each component interacts, allowing you to refine your approach and minimize risk in the live arena.
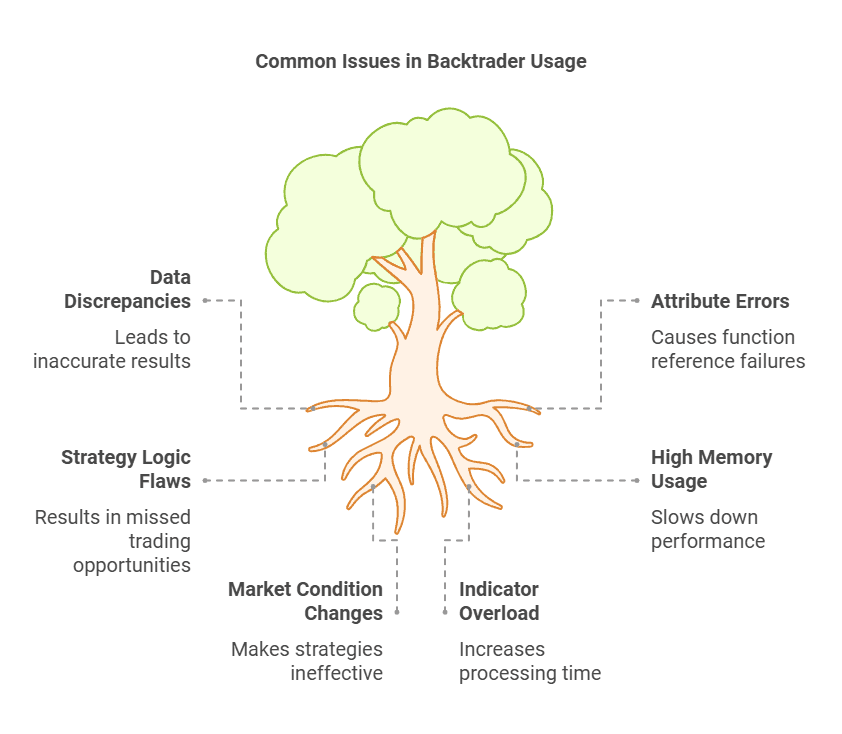
Moving from Backtesting to Live Trading with Backtrader
Many traders aspire to run their reusable trading strategies in actual market environments. Backtrader simplifies this transition by letting you shift from the backtesting process to real-time execution. But before you trade with real capital, you should confirm your algorithmic trading strategies hold up under various market conditions.
Choosing a Broker or Data Provider
Backtrader supports direct integrations with Interactive Brokers and other platforms. These links let you stream live data and place trades directly from your Python code. Remember to:
- Validate Connection Settings: Make sure you have the correct API credentials.
- Test Paper Trading: Start with a demo account if possible. This helps prevent big financial losses due to minor code errors.
Adjusting Your Strategy for Live Markets
Real-world trading introduces slippage, latency, and potential liquidity constraints. A profitable strategy in simulation might yield subpar returns once you account for these factors. To accommodate real markets, consider:
- Slippage and Commission Models: Build them into your code to simulate real trading costs.
- Dynamic Position Sizing: Limit your risk based on equity changes, especially during high volatility.
- Adaptive Indicators: Some technical indicators might need tuning for shorter timeframes or heavier volume scenarios.
Tracking Live Performance
After you go live, continue to monitor key metrics like maximum drawdown and net gains. You can set up notifications to identify unusual performance drops quickly. If your results deviate too far from your backtesting data, reevaluate your exit strategy and order types.
Balancing Automation and Oversight
Automated algorithmic traders sometimes run 24/7. Despite this convenience, you must keep an eye on trades. A sudden market event or broker outage could destabilize your positions. Periodically pause the system to:
- Check your logs for errors or big slippage spikes.
- Compare the visual plots from your live results to your historical backtests.
- Confirm that your open positions match your intended risk thresholds.
By combining thorough strategy evaluation with cautious deployment, you can integrate backtrader into a real-world workflow. Over time, you’ll gain confidence and refine every aspect of strategy development, ensuring both consistency and flexibility.
Yes. Backtrader continues to receive support through community contributions, and you can find active discussions on its GitHub repository. While major updates appear less frequently now, the project remains functional for most trading needs.
Backtrader is widely trusted by many traders because it has a stable codebase and an event-driven design. Users often praise its versatility for backtesting and live trading scenarios.
“Best” is subjective, but Backtrader ranks high among popular solutions like PyAlgoTrade and Zipline. Each tool has pros and cons. You should test them and pick the one that suits your goals and coding style.
Backtrader2 is a community-led fork aiming to fix bugs and add features beyond the original Backtrader. However, the primary Backtrader library remains robust for most traders. If you want new features sooner, you could explore Backtrader2, but it may have fewer documented resources.
No. Official support for Python 2 ended in 2020. Python 3 is the recommended version for using Backtrader and most modern Python libraries.
Yes. Backtesting can be free if you use open-source frameworks like Backtrader and free data sources. However, some premium data or specialized platforms may charge fees.
Most mid-range CPUs handle backtesting effectively when paired with enough RAM. If you plan to run large-scale or complex tests, a CPU with multiple cores and high clock speeds will help shorten computation times.
Conclusion
Backtrader is a powerful and flexible Python library that can guide you toward a more consistent and informed trading approach. Before you jump into the market, make sure you have a solid plan and are ready to adapt. Small tweaks in your entry, exit, or indicator choices can make a big difference in long-term results.
Key Takeaways
- Consistency in testing builds confidence in your trading strategy.
- Tracking performance metrics and key indicators reveals areas for improvement.
- A robust exit strategy limits losses and protects gains during market volatility.
- Careful alignment of data and time periods avoids misleading backtest outcomes.
- Always keep learning, iterating, and refining to stay ahead in shifting markets.
Looking for supercharge your algo-trading skills ?
Learn 3x faster with the TradeSearcher quiz that pinpoints the types of approaches that suit your risk tolerance and style. You can also browse our TradeSearcher database to discover real-world backtesting data and proven strategy setups. By combining the insights from your backtrader experience with these resources, you’ll find a path that aligns with your trading ambitions.